Angular – Fundamental development steps: create a new (default) component and use it
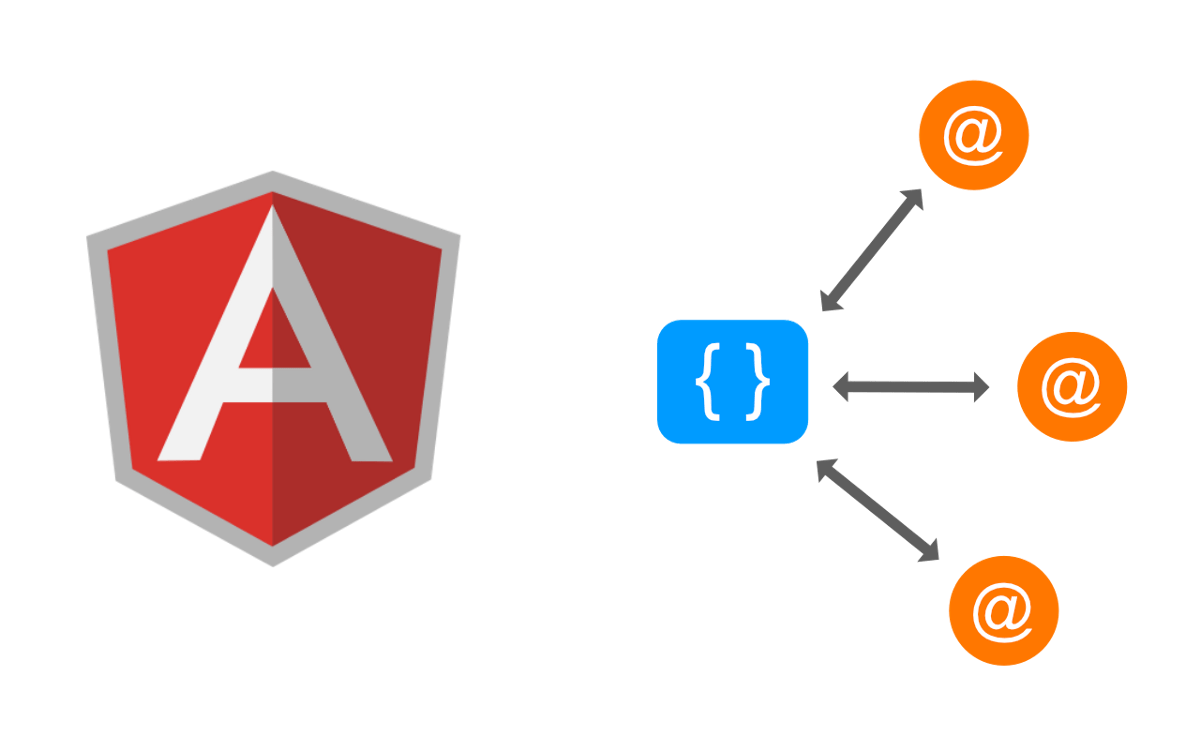
210226
In a previous document/post (Angular – Installation on Ubuntu (Kubuntu), create/start a new (default) project, and start working with) we have created a very first Project/App named Angularproj2 in a Kubuntu machine. We used the standard Angular ng command: ng new angularproj2 from within the ‘/home/panosku/angularproj1’ folder. So, the full path-name of that project root folder is located at: ‘/home/panosku/angularproj1/angularproj2‘
All the project’s source files created, are located under the /src subfolder.
panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ tree src -F src |-- app/ | |-- app-routing.module.ts | |-- app.component.css | |-- app.component.html | |-- app.component.spec.ts | |-- app.component.ts | `-- app.module.ts |-- assets/ |-- environments/ | |-- environment.prod.ts | `-- environment.ts |-- favicon.ico |-- index.html |-- main.ts |-- polyfills.ts |-- styles.css `-- test.ts 3 directories, 14 files panosku@panosku-MS-7B18:~/angularproj1/angularproj2$
When we run our application using the ng serve –open (or ng serve –host 0.0.0.0) command, we got the result at www.localhost:4200 in our browser:
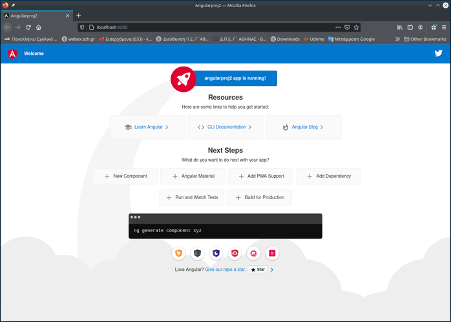
What we see above is mainly defined inside the /src/app/app.component.html file:
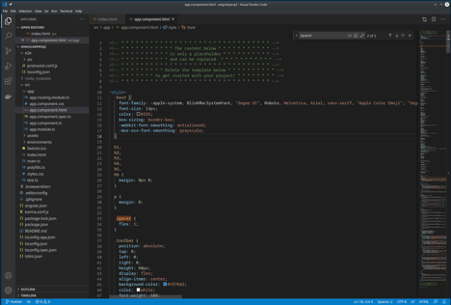
Navigating inside the app.component.html file (e.g., using the VS Code) you will notice that its content is nothing but a demo, which provides into the browser’s window some vital info about Angular framework and what some next steps can be. As it stated (inside it), whenever we want, we can remove/replace it with our custom code. (we will come back to it later and we will add a custom component of ours).
Next, let’s take a look at our project’s/app’s index.html. The index.html file is located under the /src sub-folder under the projet’s root folder (in our case its full pathname is ‘/home/panosku/angularproj1/angularproj2/index.html’)
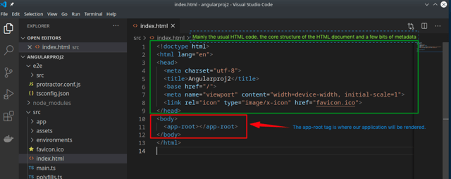
Mainly It consists of pure html code. For Angular, the important part is the:
<body> <app-root></app-root> </body>
The app-root tag is where our application will be rendered. In Angular, we can define our own HTML tags and give them custom functionality. The app-root tag will be the “entry point” for our application on the page. Actually, app-root is a component that has been defined by our Angular application.
Components
In Angular, the concept of component is one of the very fundamental ideas. Actually, Components are the most basic UI building blocks of an Angular app. In the official documentation is stated that “A component controls a patch of the screen called a view.” A Component encapsulates all: the data, the logic, and the HTML template for a view – which means everything a user sees on a particular part of the browser’s screen (controlled by the Component). Components can be used as custom tags in HTML code. In fact, with components, we inform the browser about new/custom tags and their custom functionality(-ies).
NB: In early versions (e.g., AngularJS 1.X), an analogous to the ‘component’ concept was the ‘directive’.
Create a new Component
To create a new component, we can use the generate command of the Angular CLI. For instance, to generate a component named ‘my-component’, we have to run the following command (from within project’s root folder): $ ng generate component my-component
panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ ng generate component my-component CREATE src/app/my-component/my-component.component.css (0 bytes) CREATE src/app/my-component/my-component.component.html (27 bytes) CREATE src/app/my-component/my-component.component.spec.ts (662 bytes) CREATE src/app/my-component/my-component.component.ts (298 bytes) UPDATE src/app/app.module.ts (497 bytes) panosku@panosku-MS-7B18:~/angularproj1/angularproj2$
As a result, a new component subfolder is created under the /src/app folder, containing all necessary component source files:
panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ tree src -F src |-- app/ | |-- app-routing.module.ts | |-- app.component.css | |-- app.component.html | |-- app.component.spec.ts | |-- app.component.ts | |-- app.module.ts | `-- my-component/ | |-- my-component.component.css | |-- my-component.component.html | |-- my-component.component.spec.ts | `-- my-component.component.ts |-- assets/ |-- environments/ | |-- environment.prod.ts | `-- environment.ts |-- favicon.ico |-- index.html |-- main.ts |-- polyfills.ts |-- styles.css `-- test.ts 4 directories, 18 files panosku@panosku-MS-7B18:~/angularproj1/angularproj2$
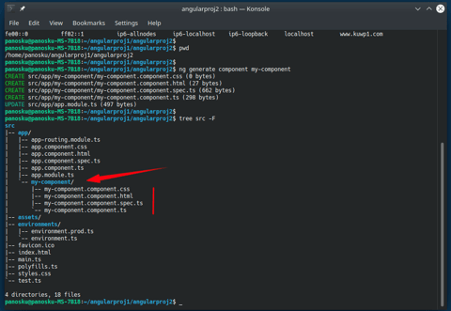
Let’s see (using VS Code) the component’s definition class. This in the my-component.component.ts typescript file. (full path-name: ‘/home/panosku/angularproj1/angularproj2/src/app/my-component/my-component.component.ts’)
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-my-component', templateUrl: './my-component.component.html', styleUrls: ['./my-component.component.css'] }) export class MyComponentComponent implements OnInit { constructor() { } ngOnInit(): void { }
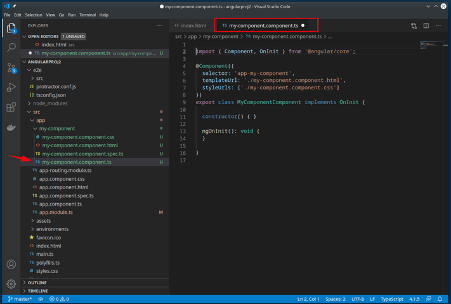
At the very beginning is the import statement. import is quite similar to import in Java. It pulls into our project all the necessary dependencies from another module and actually, it makes these dependencies available for use in our component’s class file (‘destructuring’).
In our case we are importing just two things: the ‘Component’, and the ‘OnInit’. The first allows us to define a component “decorating” it with @Componenr annotation, and including the necessary code between ({ … }) brackets. The second allows us to run the code just during the component initialization.
The first part of code (the code snippet below), is this which actually defines our component tag (named here as ‘app-my-component’), as well as its html template (my-component.component.html) and its appearance (my-component.component.css)
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-my-component', templateUrl: './my-component.component.html', styleUrls: ['./my-component.component.css'] })
This is what it is called as the component’s “decorator”.
The selector property defines which DOM element this component is going to use. In our case, any <app-my-component></ app-my-component > tags that appear within a template will have any functionality(-ies) defined within the component class.
We can define templates, either by using the template keyword in our @Component object or by specifying a templateUrl. Above we use an external file with templateUrl keyword which points to the template file: ‘my-component.component.htm’ (within the same sub-folder location).
In our case the template file: ‘my-component.component.htm’ already contains just a paragraph with some text:
<p> my-component works! </p>
(which was the default result of the ng generate component my-component command)
Alternatively, instead of using an external template file we can add a template inside our @Component by passing the template keyword option:
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-my-component', //templateUrl: './my-component.component.html', templare: ` <p> my-component works! </p> ` styleUrls: ['./my-component.component.css'] }) . . .
NB: We define our template string between backticks (` … `), which allows us to have multiline strings.
Similarly, we can have external style sheets defined, and since we can have more than one of them, we have to use an array [‘. . .’, ‘. . .’, ‘. . .’, ,..] with their names. In our case we use just one style sheet, the ‘my-component.component.css’ (within the same sub-folder location). In our case the ‘my-component.component.css’ file contains nothing (However we can add some style, e.g., color, bold text, etc. for our paragraph text in template file).
The second part of code (the code snippet below), is the definition (export) of the component class, which in our case is named ‘MyComponentComponent’ and which, similarly to Java, implement the ‘OnInit’ typescript interface. Again, in Java terms and similar to Java, there is a class constructor and a method ‘ngOnInit’ (a ‘hook’ in typescript terms) because it implements the ‘OnInit’ interface.
. . . export class MyComponentComponent implements OnInit { constructor() { } ngOnInit(): void { } }
NB: In Angular, the constructor should only be responsible for dependency injection.
Every component has a lifecycle. The ngOnInit() method is called immediately after Angular finishes setting up the component. The ngOnInit() method is the right place to put some initial operations like fetching initial data, or something we want to see immediately on page load. It is not recommended to do these kinds of operations inside the constructor, so we have ngOnInit instead.
Using / loading the newly created Component
The index.html is the root of our web page. It contains the <my-app></my-app> tag which points to the root of our angular app, i.e. to our app.component.html.
Previously we have seen the app.component.html file. The app.component.html isthe root of entire Angular app and the right place to load our newly created component. So, we can add our component somewhere inside the app.component.html. For instance we can add it between the <!– Highlight Card –> and <!– Resources –> sections:
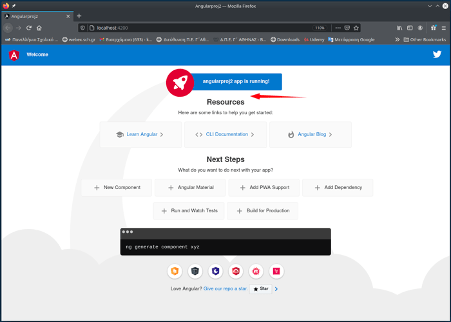
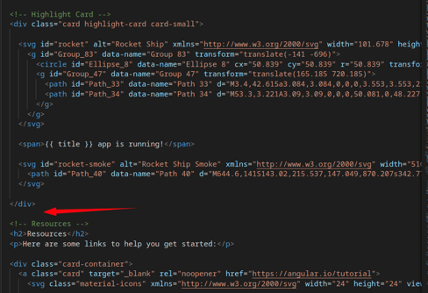
We can add the following code snippet there:
<!-- My 1st Custom Component --> <h1> <app-my-component></app-my-component> </h1>
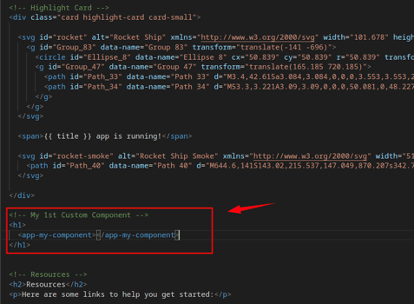
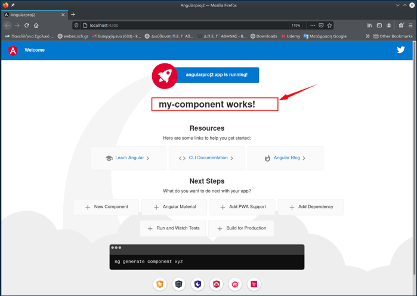
That’s it!
Thank you for reading!