Angular – Installation on Ubuntu (Kubuntu), create/start a new (default) project, and start working with
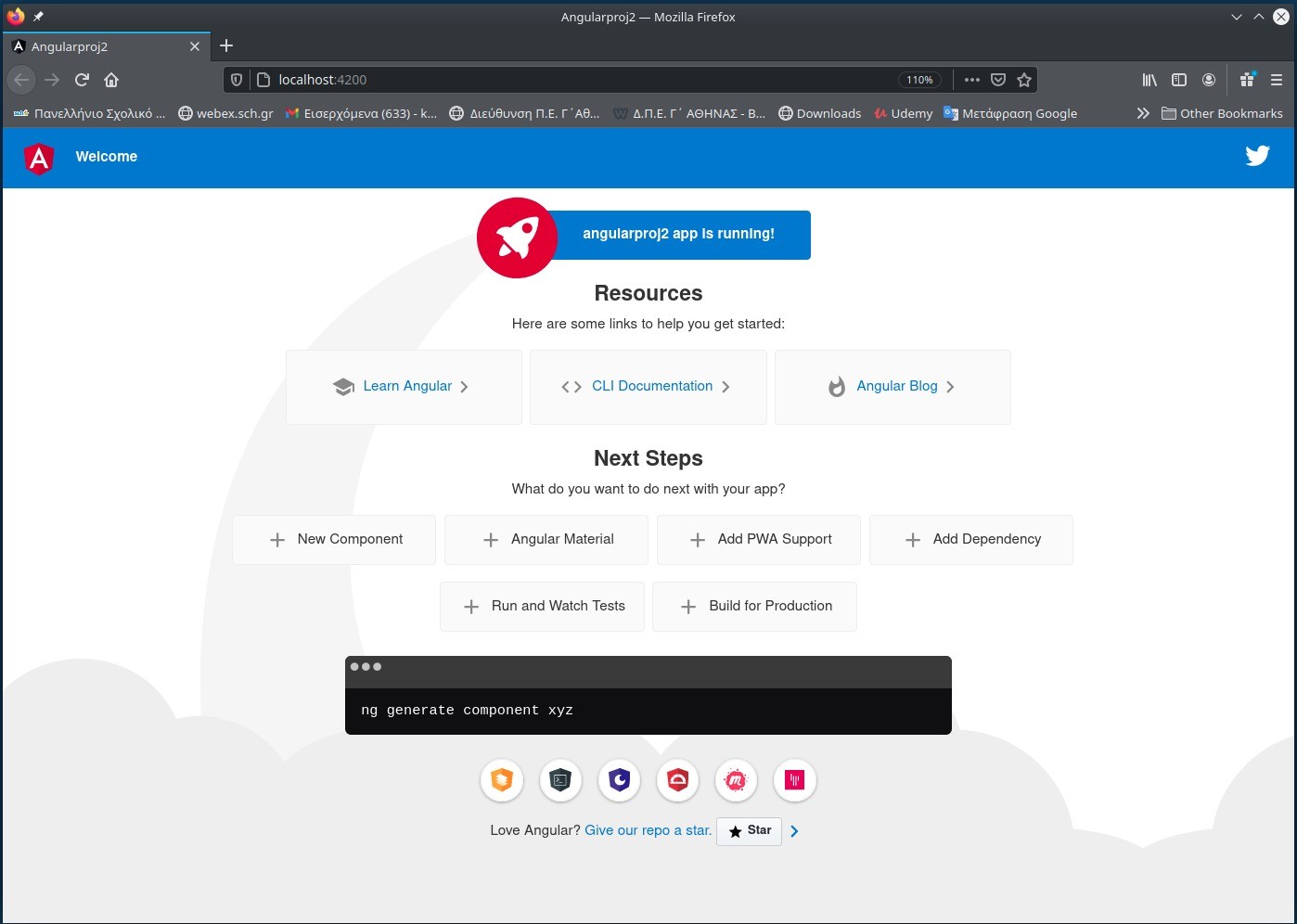
210225
Prerequisites
node
node is the core JavaScript runtime environment that runs on server-side and lets you implement your application back-end in JavaScript. Node is an Open Source Platform mainly written in C/C++. The node consists of core integrated modules, e.g., the HTTP module, in order to host a web server and other modules that can be installed via npm.
npm
npm is the Node package manager. It comes along automatically if Node is installed. All server page packages are called up and installed over npm. npm can also supply further tools.
Typescript
TypeScript is a programming language developed and maintained by Microsoft. It is a strict syntactical superset of JavaScript and adds optional static typing to the language. TypeScript is designed for the development of large applications and transcompiles to JavaScript. As TypeScript is a superset of JavaScript, existing JavaScript programs are also valid TypeScript programs.
Even if we don’t strictly have to install/use TypeScript to use Angular, installing and using it, seems to be a ‘de facto’ standard in developing Angular applications. (Angular is written in Typescript and it makes working with Angular easier).
Angular/CLI
Angular CLI is a command-line interface (CLI) to automate your development workflow like creating projects, adding new controllers, etc. It allows you to:
-create a new Angular application
-run a development server with LiveReload support to preview your application during development
-add features to your existing Angular application
-run your application’s unit tests
-run your application’s end-to-end (E2E) tests
-build your application for deployment to production
Installation on an Ubuntu/Kubuntu machine
Installation of npm and node (global installation: -g)
We can use the standard apt-get command. This will also install a number of many other dependent packages on our system.
panosku@panosku-MS-7B18:~$ panosku@panosku-MS-7B18:~$ sudo apt install nodejs . . . panosku@panosku-MS-7B18:~$ sudo apt install npm . . . panosku@panosku-MS-7B18:~$
Next we can check versions installed:
panosku@panosku-MS-7B18:~$ panosku@panosku-MS-7B18:~$ panosku@panosku-MS-7B18:~$ npm -v 6.14.4 panosku@panosku-MS-7B18:~$ panosku@panosku-MS-7B18:~$ node -v v10.19.0 panosku@panosku-MS-7B18:~$ panosku@panosku-MS-7B18:~$
Installation of Typescript (global installation: -g)
To install a third-party package in nodejs, we need “npm install” command. By this command, the nodejs will install a package in your current working directory under node_modules.
All packages contain a root file called package.json. This file contains relevant metadata about working project. This file gives information to npm that allows identifying the project’s dependencies. It’s normally located in the root directory.
npm install –g command will install third-party package in a global location for your system. You can install the global package from anywhere, no need to run this command from the current working directory. Package store in global location, we can use in any project. Global packages are for instance: angularCLI, typescript, Nodemon and etc . By this command, package.json file will not change.
panosku@panosku-MS-7B18:~$ panosku@panosku-MS-7B18:~$ sudo npm install -g typescript [sudo] password for panosku: /usr/local/bin/tsserver -> /usr/local/lib/node_modules/typescript/bin/tsserver /usr/local/bin/tsc -> /usr/local/lib/node_modules/typescript/bin/tsc + typescript@4.2.2 added 1 package from 1 contributor in 2.949s panosku@panosku-MS-7B18:~$ panosku@panosku-MS-7B18:~$
To check the TypeScript compiler version type: tsc –version
panosku@panosku-MS-7B18:~$ panosku@panosku-MS-7B18:~$ sudo tsc --version Version 4.2.2 panosku@panosku-MS-7B18:~$
Installation of Angular CLI (global installation: -g)
Again use: sudo npm install -g @angular/cli
panosku@panosku-MS-7B18:~$ panosku@panosku-MS-7B18:~$ sudo npm install -g @angular/cli npm WARN deprecated request@2.88.2: request has been deprecated, see https://github.com/request/request/issues/3142 npm WARN deprecated har-validator@5.1.5: this library is no longer supported /usr/local/bin/ng -> /usr/local/lib/node_modules/@angular/cli/bin/ng > @angular/cli@11.2.1 postinstall /usr/local/lib/node_modules/@angular/cli > node ./bin/postinstall/script.js panosku@panosku-MS-7B18:~$ panosku@panosku-MS-7B18:~$ ng version _ _ ____ _ ___ / \ _ __ __ _ _ _| | __ _ _ __ / ___| | |_ _| / △ \ | '_ \ / _` | | | | |/ _` | '__| | | | | | | / ___ \| | | | (_| | |_| | | (_| | | | |___| |___ | | /_/ \_\_| |_|\__, |\__,_|_|\__,_|_| \____|_____|___| |___/ Angular CLI: 11.2.1 Node: 10.19.0 OS: linux x64 Angular: ... Ivy Workspace: Package Version ------------------------------------------------------ @angular-devkit/architect 0.1102.1 (cli-only) @angular-devkit/core 11.2.1 (cli-only) @angular-devkit/schematics 11.2.1 (cli-only) @schematics/angular 11.2.1 (cli-only) @schematics/update 0.1102.1 (cli-only) panosku@panosku-MS-7B18:~$
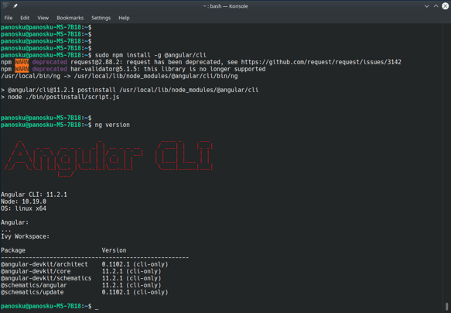
Create a new Project / Application
In order to create a new (default) Angular project (application), we can use the ng new command followed by the project’s name, e.g.: ng new angularproj2
panosku@panosku-MS-7B18:~/angularproj1$ panosku@panosku-MS-7B18:~/angularproj1$ ng new angularproj2 panosku@panosku-MS-7B18:~/angularproj1$ panosku@panosku-MS-7B18:~/angularproj1$ ls -al total 492 drwxrwxr-x 4 panosku panosku 4096 Feb 24 18:37 . drwxr-xr-x 56 panosku panosku 4096 Feb 24 18:17 .. drwxrwxr-x 6 panosku panosku 4096 Feb 24 18:37 angularproj2 drwxrwxr-x 722 panosku panosku 24576 Feb 24 18:27 node_modules -rw-rw-r-- 1 panosku panosku 462976 Feb 24 18:27 package-lock.json panosku@panosku-MS-7B18:~/angularproj1$ cd angularproj2 panosku@panosku-MS-7B18:~/angularproj1/angularproj2$
Next, let’s see the file/folder structure created:
panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ ls -al total 664 drwxrwxr-x 6 panosku panosku 4096 Feb 24 18:37 . drwxrwxr-x 4 panosku panosku 4096 Feb 24 18:37 .. -rw-rw-r-- 1 panosku panosku 703 Feb 24 18:37 .browserslistrc -rw-rw-r-- 1 panosku panosku 274 Feb 24 18:37 .editorconfig drwxrwxr-x 8 panosku panosku 4096 Feb 24 18:37 .git -rw-rw-r-- 1 panosku panosku 631 Feb 24 18:37 .gitignore -rw-rw-r-- 1 panosku panosku 1021 Feb 24 18:37 README.md -rw-rw-r-- 1 panosku panosku 3671 Feb 24 18:37 angular.json drwxrwxr-x 3 panosku panosku 4096 Feb 24 18:37 e2e -rw-rw-r-- 1 panosku panosku 1429 Feb 24 18:37 karma.conf.js drwxrwxr-x 870 panosku panosku 32768 Feb 24 18:37 node_modules -rw-rw-r-- 1 panosku panosku 581190 Feb 24 18:37 package-lock.json -rw-rw-r-- 1 panosku panosku 1202 Feb 24 18:37 package.json drwxrwxr-x 5 panosku panosku 4096 Feb 24 18:37 src -rw-rw-r-- 1 panosku panosku 287 Feb 24 18:37 tsconfig.app.json -rw-rw-r-- 1 panosku panosku 783 Feb 24 18:37 tsconfig.json -rw-rw-r-- 1 panosku panosku 333 Feb 24 18:37 tsconfig.spec.json -rw-rw-r-- 1 panosku panosku 3185 Feb 24 18:37 tslint.json panosku@panosku-MS-7B18:~/angularproj1/angularproj2$
As you can see the Git version manager is installed by default ( .git ), as well as all the source project files are located under the /src sub-folder:
panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ tree -L 1 . |-- README.md |-- angular.json |-- e2e |-- karma.conf.js |-- node_modules |-- package-lock.json |-- package.json |-- src |-- tsconfig.app.json |-- tsconfig.json |-- tsconfig.spec.json `-- tslint.json 3 directories, 9 files panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ panosku@panosku-MS-7B18:~/angularproj1/angularproj2$
Below is a short info about each one of the root level files/folders:
README.md : our project’s/app’s README text file
angular.json : the angular-cli configuration file
e2e/ : the sub-folder of end-to-end tests
node_modules/ : the sub-folder with all project’s installed dependencies
package-lock.json : npm dependencies lockfile
package.json : the npm configuration file
src/ : our project’s source code sub-folder
tsconfig.json : the typescript configuration file tslint.json : the linting configuration file
You can access the related official documentation at ‘Workspace and project file structure‘: https://angular.io/guide/file-structure
Now, let’s check again versions of packages installed for the specific project (inside the project’s root folder) using the ‘ng version’ command:
panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ ng version _ _ ____ _ ___ / \ _ __ __ _ _ _| | __ _ _ __ / ___| | |_ _| / △ \ | '_ \ / _` | | | | |/ _` | '__| | | | | | | / ___ \| | | | (_| | |_| | | (_| | | | |___| |___ | | /_/ \_\_| |_|\__, |\__,_|_|\__,_|_| \____|_____|___| |___/ Angular CLI: 11.2.1 Node: 10.19.0 OS: linux x64 Angular: 11.2.2 ... animations, common, compiler, compiler-cli, core, forms ... platform-browser, platform-browser-dynamic, router Ivy Workspace: Yes Package Version --------------------------------------------------------- @angular-devkit/architect 0.1102.1 @angular-devkit/build-angular 0.1102.1 @angular-devkit/core 11.2.1 @angular-devkit/schematics 11.2.1 @angular/cli 11.2.1 @schematics/angular 11.2.1 @schematics/update 0.1102.1 rxjs 6.6.3 typescript 4.1.5 panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ panosku@panosku-MS-7B18:~/angularproj1/angularproj2$
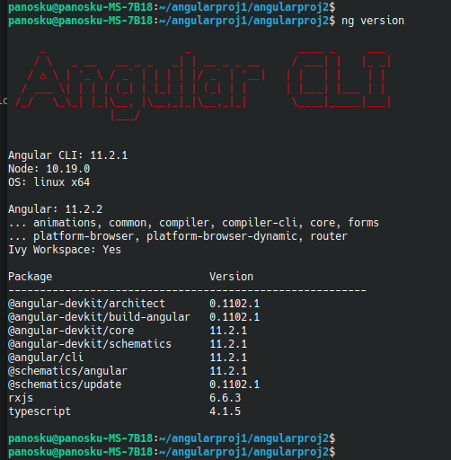
NB: As you can see above, asynchronous RxJS has been also installed (in our case this is version 6.6.3)
Now, we can run the new application by using the command: ng serve –open
panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ ng serve --open Compiling @angular/core : es2015 as esm2015 Compiling @angular/common : es2015 as esm2015 Compiling @angular/platform-browser : es2015 as esm2015 Compiling @angular/router : es2015 as esm2015 Compiling @angular/platform-browser-dynamic : es2015 as esm2015 ✔ Browser application bundle generation complete. Initial Chunk Files | Names | Size vendor.js | vendor | 2.69 MB polyfills.js | polyfills | 128.76 kB main.js | main | 58.04 kB runtime.js | runtime | 6.15 kB styles.css | styles | 119 bytes | Initial Total | 2.87 MB Build at: 2021-02-24T16:45:35.085Z - Hash: 69a72351c4c66da1bf47 - Time: 11226ms ** Angular Live Development Server is listening on localhost:4200, open your browser on http://localhost:4200/ ** ✔ Compiled successfully. ✔ Browser application bundle generation complete. Initial Chunk Files | Names | Size styles.css | styles | 119 bytes 4 unchanged chunks Build at: 2021-02-24T16:45:35.574Z - Hash: 8e126e1b62f93c60f0dd - Time: 209ms ✔ Compiled successfully.
Starting the application using the ‘ng serve –open’ command, also automatically opens a (default) browser’s window pointing to localhost port:4200:
www.localhost:4200
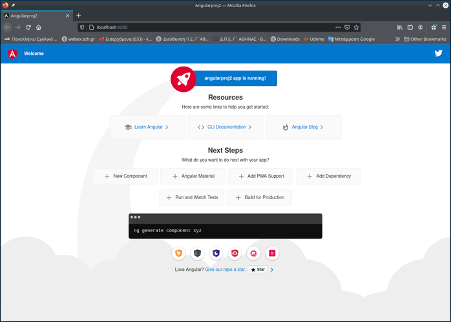
NB: In case you got a message like: ‘Port 4200 is already in use. Use ‘–port’ to specify a different port’, then it means that you have to select another port (e.g. port 9001) and re-start the app using the –port flag with ng serve command like that: ng serve –port 9001
Accessing the Angular application from another host.
If you want your Angular application to be accessible from any other host in your local network you have to use the –host parameter with 0.0.0.0 as ip, like that: $ ng serve –host 0.0.0.0
Be aware that Angular will warn you about the fact that this is not a real production server and you should be careful:
“This is a simple server for use in testing or debugging Angular applications locally. It hasn’t been reviewed for security issues. Binding this server to an open connection can result in compromising your application or computer. Using a different host than the one passed to the “–host” flag might result in websocket connection issues. You might need to use “–disableHostCheck” if that’s the case.”
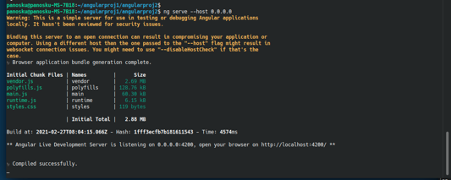
In most of the cases and for development / testing purposes, you can ignore the warnings.
After that you will be able to access it from another host by using the IP of the (Kubuntu) machine running your Angular application. e.g. www.192.168.0.84:4200
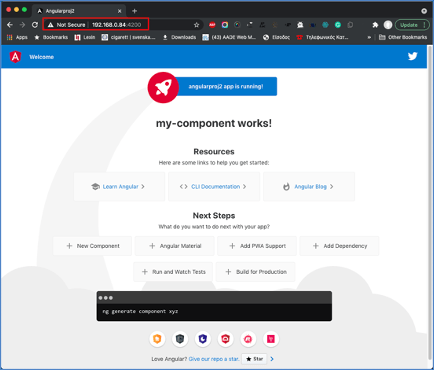
Angular source Project (Application) folder/file structure – Start working on.
We said before, that all the source code of our project is located under the src sub-folder:
panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ tree src -F src |-- app/ | |-- app-routing.module.ts | |-- app.component.css | |-- app.component.html | |-- app.component.spec.ts | |-- app.component.ts | `-- app.module.ts |-- assets/ |-- environments/ | |-- environment.prod.ts | `-- environment.ts |-- favicon.ico |-- index.html |-- main.ts |-- polyfills.ts |-- styles.css `-- test.ts 3 directories, 14 files panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ panosku@panosku-MS-7B18:~/angularproj1/angularproj2$
Now you are ready to start working with your project, e.g. you can use VS Code to start working with your code:
panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ panosku@panosku-MS-7B18:~/angularproj1/angularproj2$ code . panosku@panosku-MS-7B18:~/angularproj1/angularproj2$
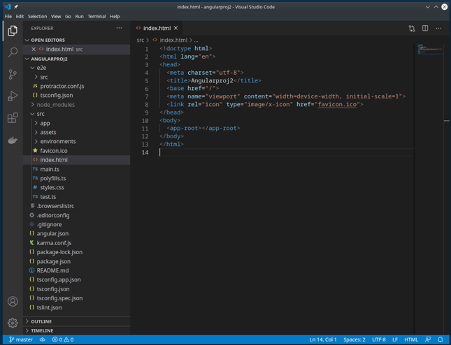
That’s it!
Thank you for reading!