Use a bash script to automate a Typescript-Node project scaffolding in your Mac
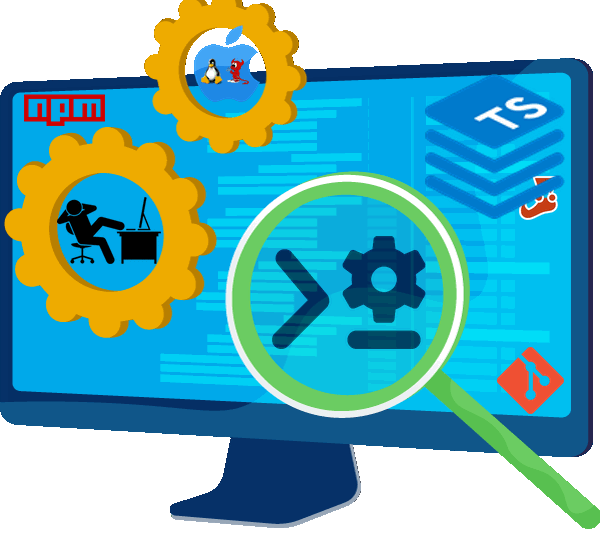
211022-1104
tl;dr
Typical steps for a project creation
Anyone can make a google or search elsewhere (e.g. in Medium) to find hundreds and hundreds of posts related to setting up a Typescript project with Node.js.
Creating a minimum possible Node/Typescript project from scratch includes a number of steps that every time we create a new project should be followed.
The very fundamental steps are:
- Create the project folder and a minimal folder structure
- Initialize the node/npm (create the project package.json file)
- Install Typescript
- Configure the TypeScript and adjust the tsconfig.json
- Install other node modules and libraries (production or development dependencies)
- Install and configure a Testing framework (e.g. Jest)
- Update the Scripts section in the package.json file, so it will be easy to compile/build, run and test the app during the development process.
- Create the project’s entry point file and some other files that might be necessary
After you start working and continue developing your project, you will see that there are also some further steps to be followed. These for example include:
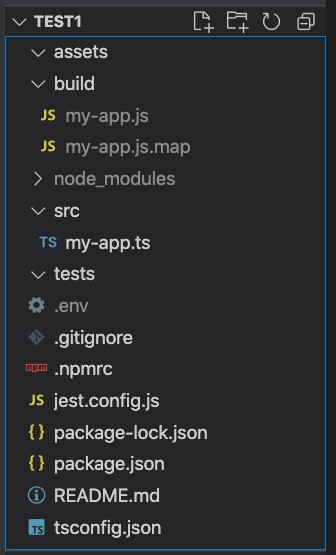
- Create the project’s .git repo (excluding unnecessary folders and files via .gitignore)
- Make an initial commit and create the main branch
- Create and push the initial branch to your remote repo
- Add a linter (e.g. ESLint) and/or code formatter (i.e. Prettier), and other ESLint plugins (e.g. a security plugin) on top of it
- Add git hooks functionality to automate a bit the push process using a
- Add a browser bundler (e.g. ESBuild)
The problem
However, even in the case you are a newcomer, sooner or later, you will start boring the routine of repeating, again and again, the same actions. After that, you will start wondering if there are possibilities to automate (all or some) all those actions and save some of your time dealing with dull stuff of the workflow: creating folders and files (scaffolding), installation of common packages, configurations, adjustments of *.json and other files, etc.
Other Solutions
There are several options you can choose to automate this process. Just few of them are:
- Play around with an external tool e.g. Grunt, Yeoman, Footsteps (an Electron-based GUI app), etc
- Use an npm package aiming to do the job, e.g.: simple-scaffold, generator-scaffold, scaffold-generator, etc.
- Follow the project template approach (using Node.js CLI)
- Keep aside a backup of your initial project setup scaffold (e.g. locally or use a remote repo), which then can be used as a basis for other projects
You can easily google and find more info about them, as well as to many other similar approaches and tools.Each one of the above options has pros and cons.
The bash script
However, here there is a quite simple approach using just a pure bash shell script. No external tools or npm packages. Just a quick, clean and fast solution, especially handy for your very fundamental installations. It doesn’t use (so far) any kind of interaction with the user (asking questions, etc), and thus, it can save enough of your routine time. No commands to give, no open-close IDEs, no open, update and save files, etc. Moreover, using it you will always have installed the recent versions of packages involved.
So, below I give you such an example script of mine, that you can use. The script is named ‘npmts.sh’.
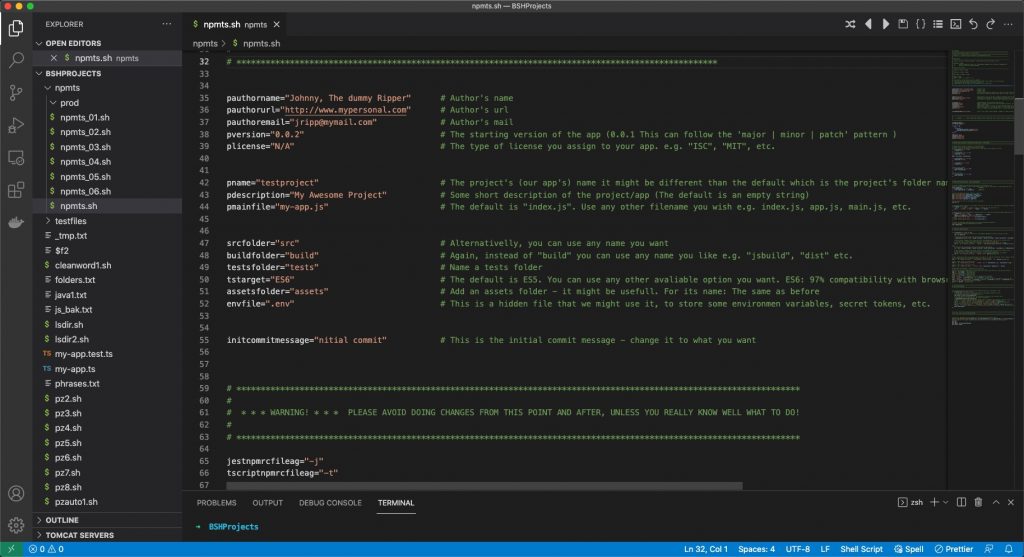
Note that this is the ‘basic’ version of the script that includes only the first 10 steps given above. An ‘augmented’ version will follow soon to cover the rest of the steps.
Script usage
You can copy the script from below, and then, put it in a file named npmtsc.sh in a folder. Give the script permission to make it executable, e.g. :
chmod u+x npmts.sh
If you wish you can copy the script to one of the folders that it is included in your $PATH, e.g.: /usr/local/bin
Run the script in your system:
<folder / path-name>npmts or <folder / path-name>npmts -t or <folder / path-name>npmts -j or <folder / path-name>npmts – t -j
Prerequisites, assumptions, and interest points
- I can’t see any point for detailed explanations for each of the tools and options are chosen here since you can easily find tons of descriptions and explanations about them. Furthermore, there are comments that at least give you some grasp of how the script works.
- The script uses very basic bash commands as well as the Linux stream editor (sed). However, this is a macOS (BSD-based) version of sed, thus, it runs on macs ***only***. (If you are familiar with ‘standard’ (e.g. GNU based) versions of sed you can make the necessary adjustments on your own).
- Node / npm should have been installed on your Mac.
- Typescript globally installed is OK. However, the script allows you also choose to install Typescript as a development dependency, locally, for your project (use the -t flag for this).
- You can use the -j flag to install the Jest testing framework, as well.
- The folder structure and the whole scaffold are installed inside the directory you already are when you run the script.
- The script creates and uses a project local .npmrc file containing the basic values that will be used for package.json parameters (options). The .npmrc file created can also be used independently for other projects of yours.
- Last but quite important! You can assign some values to variables being used in the script which suit you. Those variables are located in lines between 20 and 40 and you can give the values you prefer. As you can see below the comments are quite descriptive and almost self-explanatory. However, please avoid making changes after line 40.
So, this is the code:
Disclaimer:
The script simply creates a project’s scaffold. However, you use and run the script at your own risk. This is especially important in case you decide to make changes on your own.
Let me know if you find problems or if there is something missing.
Enjoy, and stay tuned.
Thanx for reading!